Control Structure
By Paribesh Sapkota
Control Structures
Control Structures are just a way to specify flow of control in programs. Any algorithm or program can be more clear and understood if they use self-contained modules called as logic or control structures. It basically analyzes and chooses in which direction a program flows based on certain parameters or conditions.
Characteristics:
- Control statements serve to control the execution flow of a program.
- Control statements in C are classified into selection statements, iteration statements, and jump statements.
- Selection statements serve to execute code based on a certain circumstance.
- Iteration statements loop through a code block until a condition is met.
- Jump statements serve to move control from one section of a program to another.
- The if statement, for loop, while loop, switch statement, break statement, and continue statement are C’s most widely used control statements.
There are three basic types of logic, or flow of control, known as:
- Sequence logic, or sequential flow
- Selection logic, or conditional flow
- Iteration logic, or repetitive flow
Sequential Logic (Sequential Flow)
Sequential logic as the name suggests follows a serial or sequential flow in which the flow depends on the series of instructions given to the computer. Unless new instructions are given, the modules are executed in the obvious sequence. The sequences may be given, by means of numbered steps explicitly. Also, implicitly follows the order in which modules are written. Most of the processing, even some complex problems, will generally follow this elementary flow pattern.
Decision Statements(Selective Control Structure)
In selective control structure, selection is made on the basis of condition. We have options to go when the given condition is true or false. The flow of program statements execution is totally directed by the result obtained from checking condition. Hence, program statements using selective control structures are also called conditional statements. It can mainly be categorized into two types.
- Conditional Statement
- Switch-Case Statement
Conditional Statement
It is the most common decision making control structure which controls the flow of program statement execution based on the condition checked. it can be used in different forms as:
- if statement
- if else statement
- if else if statement (Multipath Conditional Statement/ if-else ladder)
- nested if else statement
if statement
This is the simplest form of conditional statement in which statements are executed if the test expression (condition) is true. When condition is false there is no option to go within this structure; in such situation control must get out from the structure and statements outside this structure will be executed.
if(expression){ //code to be executed }
Flowchart of if statement in C
#include<stdio.h> int main(){ int number=0; printf("Enter a number:"); scanf("%d",&number); if(number%2==0){ printf("%d is even number",number); } return 0; }
Output
Enter a number:4 4 is even number enter a number:5
Program to find the largest number of the three.
#include <stdio.h> int main() { int a, b, c; printf("Enter three numbers?"); scanf("%d %d %d",&a,&b,&c); if(a>b && a>c) { printf("%d is largest",a); } if(b>a && b > c) { printf("%d is largest",b); } if(c>a && c>b) { printf("%d is largest",c); } if(a == b && a == c) { printf("All are equal"); } }
Output
Enter three numbers? 12 23 34 34 is largest
If-else Statement
The if-else statement is a conditional statement used for decision-making. It allows us to execute different blocks of code based on whether a certain condition evaluates to true or false. The basic syntax of the if-else statement is as follows:
if (condition) { // Code block to be executed if the condition is true } else { // Code block to be executed if the condition is false }
Here’s a breakdown of how it works:
- The
condition
is an expression that evaluates to either true or false. - If the
condition
is true, the code block immediately following theif
statement is executed. - If the
condition
is false, the code block immediately following theelse
statement is executed. - The
else
block is optional. If it’s not included, the program will simply skip the code block associated with theelse
statement if theif
condition evaluates to false.
Flowchart of the if-else statement in C
#include<stdio.h> int main(){ int number=0; printf("enter a number:"); scanf("%d",&number); if(number%2==0){ printf("%d is even number",number); } else{ printf("%d is odd number",number); } return 0; }
Output
enter a number:4 4 is even number enter a number:5 5 is odd number
Program to check whether a person is eligible to vote or not.
#include <stdio.h> int main() { int age; printf("Enter your age?"); scanf("%d",&age); if(age>=18) { printf("You are eligible to vote..."); } else { printf("Sorry ... you can't vote"); } }
Output
Enter your age?18 You are eligible to vote... Enter your age?13 Sorry ... you can't vote example
#include <stdio.h> int main() { int num = 10; if (num > 0) { printf("%d is positive.\n", num); } else { printf("%d is not positive.\n", num); } return 0; }
In this example:
- The condition
num > 0
checks if the variablenum
is greater than 0. - If the condition is true (which it is because
num
is 10), the program executes the code block inside theif
statement, printing that10 is positive
. - If the condition is false, the program executes the code block inside the
else
statement (which in this case doesn’t happen).
if else if Statement
The if-else if statement is an extension of the basic if-else statement. It allows you to test multiple conditions and execute different blocks of code based on which condition evaluates to true first. This construct is particularly useful when you have multiple conditions to consider.
The syntax of the if-else if statement is as follows:
if (condition1) { // Code block to be executed if condition1 is true } else if (condition2) { // Code block to be executed if condition2 is true } else if (condition3) { // Code block to be executed if condition3 is true } ... else { // Code block to be executed if none of the above conditions are true }
Here’s how it works:
- The program evaluates each condition in sequence until one of them is true.
- If a condition evaluates to true, the corresponding code block is executed, and the program skips the remaining conditions.
- If none of the conditions evaluate to true, the code block associated with the
else
statement (if present) is executed.
Here’s an example:
#include <stdio.h> int main() { int num = 10; if (num > 0) { printf("%d is positive.\n", num); } else if (num < 0) { printf("%d is negative.\n", num); } else { printf("%d is zero.\n", num); } return 0; }
In this example:
- The first condition
num > 0
checks if the variablenum
is greater than 0. - If this condition is true, the program prints that
10 is positive
. - If the first condition is false, the program checks the next condition
num < 0
, which checks ifnum
is less than 0. - If this condition is true, the program prints that
10 is negative
. - If neither of the conditions is true (which is not the case here), the program executes the code block associated with the
else
statement, printing that10 is zero
.
Program to calculate the grade of the student according to the specified marks.
#include <stdio.h> int main() { int marks; printf("Enter your marks?"); scanf("%d",&marks); if(marks > 85 && marks <= 100) { printf("Congrats ! you scored grade A ..."); } else if (marks > 60 && marks <= 85) { printf("You scored grade B + ..."); } else if (marks > 40 && marks <= 60) { printf("You scored grade B ..."); } else if (marks > 30 && marks <= 40) { printf("You scored grade C ..."); } else { printf("Sorry you are fail ..."); } }
Output
Enter your marks?10 Sorry you are fail ... Enter your marks?40 You scored grade C ... Enter your marks?90 Congrats ! you scored grade A ...
Nested if-else in C
A nested if in C is an if statement that is the target of another if statement. Nested if statements mean an if statement inside another if statement. Yes, C allow us to nested if statements within if statements, i.e, we can place an if statement inside another if statement.
Syntax of Nested if-else
if (condition1) { // Executes when condition1 is true if (condition2) { // Executes when condition2 is true } else { // Executes when condition2 is false }
Flowchart of Nested if-else
Example of Nested if-else
#include <stdio.h> int main() { int num1 = 10; int num2 = 5; if (num1 > 0) { if (num2 > 0) { printf("Both numbers are positive.\n"); } else { printf("Num1 is positive, but num2 is not.\n"); } } else { if (num2 > 0) { printf("Num2 is positive, but num1 is not.\n"); } else { printf("Both numbers are non-positive.\n"); } } return 0; }
In this example:
- We have two variables
num1
andnum2
with values 10 and 5, respectively. - We use nested if-else statements to check the positivity of both numbers.
- If
num1
is positive (num1 > 0
), we enter the inner if-else statement to check the positivity ofnum2
. - If both
num1
andnum2
are positive, it prints “Both numbers are positive.” - If
num1
is positive butnum2
is not, it prints “Num1 is positive, but num2 is not.” - If
num1
is not positive (num1 <= 0
), we enter the else block of the outer if statement, and similar checks are performed fornum2
. - Finally, if neither
num1
nornum2
is positive, it prints “Both numbers are non-positive.”
C Switch Statement
switch statement is a control flow statement used to select one of many code blocks to be executed based on the value of a variable or an expression. It provides an alternative way to express multiple conditional branches compared to using multiple if-else if-else statements. The switch statement is particularly useful when you have a large number of conditions to evaluate.
The basic syntax of the switch statement is as follows:
switch (expression) { case constant1: // Code block to be executed if expression equals constant1 break; case constant2: // Code block to be executed if expression equals constant2 break; // More case statements as needed default: // Code block to be executed if expression doesn't match any case constant }
Here’s a breakdown of how it works:
- The
expression
is evaluated once and its value is compared with the values of thecase
constants. - If a match is found between the
expression
and acase
constant, the code block associated with thatcase
label is executed. - The
break
statement terminates the switch statement. Without it, control falls through to the next case label. This behavior allows for executing multiple cases together. - If no match is found between the
expression
and anycase
constant, the code block associated with thedefault
label (if present) is executed. Thedefault
case is optional.
Flowchart of switch statement in C
Example of a switch statement in C
#include<stdio.h> int main(){ int number=0; printf("enter a number:"); scanf("%d",&number); switch(number){ case 10: printf("number is equals to 10"); break; case 50: printf("number is equal to 50"); break; case 100: printf("number is equal to 100"); break; default: printf("number is not equal to 10, 50 or 100"); } return 0; }
Output
enter a number:4 number is not equal to 10, 50 or 100 enter a number:50 number is equal to 50
Break and Default keyword in Switch statement
Let us explain and define the “break” and “default” keywords in the context of the switch statement, along with example code and output.
1. Break Keyword:
The “break” keyword is used within the code block of each case to terminate the switch statement prematurely. When the program encounters a “break” statement inside a case block, it immediately exits the switch statement, preventing the execution of subsequent case blocks. The “break” statement is crucial for avoiding switch statements’ “fall-through” behavior.
Example:
Let’s take a program to understand the use of the break keyword in C.
#include <stdio.h> int main() { int num = 3; switch (num) { case 1: printf("Value is 1\n"); break; // Exit the switch statement after executing this case block case 2: printf("Value is 2\n"); break; // Exit the switch statement after executing this case block case 3: printf("Value is 3\n"); break; // Exit the switch statement after executing this case block default: printf("Value is not 1, 2, or 3\n"); break; // Exit the switch statement after executing the default case block } return 0; }
Output
Value is 3
Explanation:
In this example, the switch statement evaluates the value of the variable num (which is 3) and matches it with case 3. The code block associated with case 3 is executed, printing “Value is 3” to the console. The “break” statement within case 3 ensures that the program exits the switch statement immediately after executing this case block, preventing the execution of any other cases.
Default Keyword:
When none of the case constants match the evaluated expression, it operates as a catch-all case. If no matching case exists and a “default” case exists, the code block associated with the “default” case is run. It is often used to handle circumstances where none of the stated situations apply to the provided input.
Example:
Let’s take a program to understand the use of the default keyword in C.
#include <stdio.h> int main() { int num = 5; switch (num) { case 1: printf("Value is 1\n"); break; case 2: printf("Value is 2\n"); break; case 3: printf("Value is 3\n"); break; default: printf("Value is not 1, 2, or 3\n"); break; // Exit the switch statement after executing the default case block } return 0; }
Output
Value is not 1, 2, or 3
Explanation:
In this example, the switch statement examines the value of the variable num (which is 5). Because no case matches the num, the program performs the code block associated with the “default” case. The “break” statement inside the “default” case ensures that the program exits the switch statement after executing the “default” case block.
Both the “break” and “default” keywords play essential roles in controlling the flow of execution within a switch statement. The “break” statement helps prevent the fall-through behavior, while the “default” case provides a way to handle unmatched cases.
Nested switch case statement
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
#include <stdio.h> int main () { int i = 10; int j = 20; switch(i) { case 10: printf("the value of i evaluated in outer switch: %d\n",i); case 20: switch(j) { case 20: printf("The value of j evaluated in nested switch: %d\n",j); } } printf("Exact value of i is : %d\n", i ); printf("Exact value of j is : %d\n", j ); return 0; }
Output
the value of i evaluated in outer switch: 10 The value of j evaluated in nested switch: 20 Exact value of i is : 10 Exact value of j is : 20
C Loops
In C programming, loops are used to execute a block of code repeatedly until a specified condition is met. There are three main types of loops in C: for
, while
, and do-while
. Each loop has its own syntax and use cases.
for Loop:
The for
loop is used when you know how many times you want to execute a block of code. It consists of three parts: initialization, condition, and increment/decrement. syntax
for (initialization; condition; increment/decrement) { // Code to be executed repeatedly }
Flowchart of for Loop
example:
wap to display number from 1 t0 10 using for loop in c
#include <stdio.h> int main() { int i; // Using a for loop to display numbers from 1 to 10 for (i = 1; i <= 10; i++) { printf("%d\n", i); } return 0; }
In this program:
- We declare an integer variable
i
to use as the loop counter. - The for loop is initialized with
i = 1
to start from 1. - The loop condition is
i <= 10
, meaning the loop will continue as long asi
is less than or equal to 10. - After each iteration,
i
is incremented by 1 (i++
). - Inside the loop, we print the value of
i
usingprintf
. - The loop continues until
i
reaches 10, and then the program terminates.
while Loop: The while
loop is used when you want to execute a block of code as long as a condition is true. It evaluates the condition before executing the block of code.
syntax
while (condition) { // Code to be executed repeatedly }
Flowchart of while loop in C
example:
- Display your name 10 times on the screen using a while loop:
#include <stdio.h> int main() { int count = 0; // Displaying the name 10 times using a while loop while (count < 10) { printf("Your Name\n"); count++; } return 0; }
2. Display the series from 10 to 1 using a while loop:
#include <stdio.h> int main() { int num = 10; // Displaying the series from 10 to 1 using a while loop while (num >= 1) { printf("%d ", num); num--; } return 0; }
3. Calculate and display the sum of odd natural numbers up to n using a while loop:
#include <stdio.h> int main() { int n, sum = 0, num = 1; printf("Enter a number: "); scanf("%d", &n); // Calculating and displaying the sum of odd natural numbers up to n while (num <= n) { sum += num; num += 2; // Incrementing num by 2 to get the next odd number } printf("Sum of odd natural numbers up to %d is: %d\n", n, sum); return 0; }
do-while Loop: The do-while
loop is similar to the while
loop, but it evaluates the condition after executing the block of code, so the block of code is guaranteed to execute at least once.
syntax
do { // Code to be executed repeatedly } while (condition);
do…while Loop Flowchart
example:
- Calculate and display the series 1,8,27,… up to the 10th term using a do-while loop:
#include <stdio.h> int main() { int term = 1; int num = 1; // Displaying the series up to the 10th term using a do-while loop do { printf("%d ", num); num = num * term; term++; } while (term <= 10); return 0; }
2. Display the series 15,9,… up to the 20th term using a do-while loop:
#include <stdio.h> int main() { int term = 1; int num = 15; // Displaying the series up to the 20th term using a do-while loop do { printf("%d ", num); num = num - 6; term++; } while (term <= 20); return 0; }
3. Display the multiplication table of any number given by the user using a do-while loop:
#include <stdio.h> int main() { int num, i = 1; printf("Enter a number: "); scanf("%d", &num); printf("Multiplication table of %d:\n", num); // Displaying the multiplication table using a do-while loop do { printf("%d x %d = %d\n", num, i, num * i); i++; } while (i <= 10); return 0; }
Continue Statement
The C continue statement resets program control to the beginning of the loop when encountered. As a result, the current iteration of the loop gets skipped and the control moves on to the next iteration. Statements after the continue statement in the loop are not executed.
Syntax of continue in C
The syntax of continue is just the continue keyword placed wherever we want in the loop body.
continue;
Use of continue in C
The continue statement in C can be used in any kind of loop to skip the current iteration. In C, we can use it in the following types of loops:
- Single Loops
- Nested Loops
Using continue in infinite loops is not useful as skipping the current iteration won’t make a difference when the number of iterations is infinite.
// C program to explain the use // of continue statement with for loop #include <stdio.h> int main() { // for loop to print 1 to 8 for ( int i = 1; i <= 8; i++) { // when i = 4, the iteration will be skipped and for // will not be printed if (i == 4) { continue ; } printf ( "%d " , i); } printf ( "\n" ); int i = 0; // while loop to print 1 to 8 while (i < 8) { // when i = 4, the iteration will be skipped and for // will not be printed i++; if (i == 4) { continue ; } printf ( "%d " , i); } return 0; } |
Output
1 2 3 5 6 7 8 1 2 3 5 6 7 8
Example 2: C Program to use continue in a nested loop
The continue statement will only work in a single loop at a time. So in the case of nested loops, we can use the continue statement to skip the current iteration of the inner loop when using nested loops.
C
// C program to explain the use // of continue statement with nested loops #include <stdio.h> int main() { // outer loop with 3 iterations for ( int i = 1; i <= 3; i++) { // inner loop to print integer 1 to 4 for ( int j = 0; j <= 4; j++) { // continue to skip printing number 3 if (j == 3) { continue ; } printf ( "%d " , j); } printf ( "\n" ); } return 0; } |
Output
0 1 2 4 0 1 2 4 0 1 2 4
Flowchart of continue in C
Break Statement
The break in C is a loop control statement that breaks out of the loop when encountered. It can be used inside loops or switch statements to bring the control out of the block. The break statement can only break out of a single loop at a time.
Syntax of break in C
break;
Use of break in C
The break statement in C is used for breaking out of the loop. We can use it with any type of loop to bring the program control out of the loop. In C, we can use the break statement in the following ways:
- Simple Loops
- Nested Loops
- Infinite Loops
- Switch case
Examples of break in C
Example 1: C Program to use break Statement with Simple Loops
Break statements in C can be used with simple loops i.e, for loops, while loops, and do-while loops.
// C Program to demonstrate break statement with for loop #include <stdio.h> int main() { // using break inside for loop to terminate after 2 // iteration printf ( "break in for loop\n" ); for ( int i = 1; i < 5; i++) { if (i == 3) { break ; } else { printf ( "%d " , i); } } // using break inside while loop to terminate after 2 // iteration printf ( "\nbreak in while loop\n" ); int i = 1; while (i < 20) { if (i == 3) break ; else printf ( "%d " , i); i++; } return 0; } |
Output
break in for loop 1 2 break in while loop 1 2
Example 2: C Program to use break Statement with Nested Loops
Break statements can also be used when working with nested loops. The control will come out of only that loop in which the break statement is used.
// C program to illustrate // using break statement // in Nested loops #include <stdio.h> int main() { // nested for loops with break statement // at inner loop for ( int i = 1; i <= 6; ++i) { for ( int j = 1; j <= i; ++j) { if (i <= 4) { printf ( "%d " , j); } else { // if i > 4 then this innermost loop will // break break ; } } printf ( "\n" ); } return 0; } |
Output
1 1 2 1 2 3 1 2 3 4
C Program to use break Statement with Infinite Loops
An Infinite loop can be terminated with a break statement as a part of the condition.
// C Program to demonstrate infinite loop without using // break statement #include <stdio.h> int main() { int i = 0; // while loop which will always be true while (1) { printf ( "%d " , i); i++; if (i == 5) { break ; } } return 0; } |
Output
0 1 2 3 4
Flowchart of break in C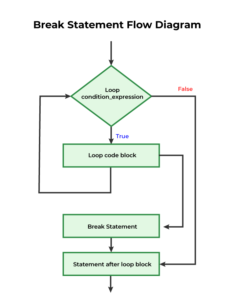
Break in C switch case
Syntax of break in switch case
switch(expression) { case value1: statement_1; break; case value2: statement_2; break; ..... ..... case value_n: statement_n; break; default: default statement; }
Example of break in switch case
// C Program to demonstrate working of break with switch // case #include <stdio.h> #include <stdlib.h> int main() { char c; float x, y; while (1) { printf ( "Enter an operator (+, -), if want to exit " "press x: " ); scanf ( " %c" , &c); // to exit if (c == 'x' ) exit (0); printf ( "Enter Two Values:\n " ); scanf ( "%f %f" , &x, &y); switch (c) { // For Addition case '+' : printf ( "%.1f + %.1f = %.1f\n" , x, y, x + y); break ; // For Subtraction case '-' : printf ( "%.1f - %.1f = %.1f\n" , x, y, x - y); break ; default : printf ( "Error! please write a valid operator\n" ); } } } |
Output:
Enter an operator (+, -), if want to exit press x: + Enter Two Values: 10 20 10.0 + 20.0 = 30.0
What is the difference between break and continue?
The difference between the break and continue in C is listed in the below table:
break | continue |
---|---|
The break statement terminates the loop and brings the program control out of the loop. | The continue statement terminates only the current iteration and continues with the next iterations. |
The syntax is:
break; |
The syntax is:
continue; |
The break can also be used in switch case. | Continue can only be used in loops. |
goto statement
The goto statement is known as jump statement in C. As the name suggests, goto is used to transfer the program control to a predefined label. The goto statment can be used to repeat some part of the code for a particular condition. It can also be used to break the multiple loops which can’t be done by using a single break statement. However, using goto is avoided these days since it makes the program less readable and complicated.
Syntax:
- label:
- //some part of the code;
- goto label;
goto example
Let’s see a simple example to use goto statement in C language.
#include <stdio.h> int main() { int num,i=1; printf("Enter the number whose table you want to print?"); scanf("%d",&num); table: printf("%d x %d = %d\n",num,i,num*i); i++; if(i<=10) goto table; }
Output:
Enter the number whose table you want to print?10 10 x 1 = 10 10 x 2 = 20 10 x 3 = 30 10 x 4 = 40 10 x 5 = 50 10 x 6 = 60 10 x 7 = 70 10 x 8 = 80 10 x 9 = 90 10 x 10 = 100
Exit Function
In C programming, the exit()
function is used to terminate the program’s execution immediately. It allows you to exit from anywhere in the program, including inside functions, loops, or nested blocks. The exit()
function takes an integer argument that represents the exit status of the program.
Here’s the syntax of the exit()
function:
#include <stdlib.h> void exit(int status);
- The
status
parameter is an integer value that represents the exit status of the program. A value of 0 typically indicates successful execution, while a non-zero value indicates an error or abnormal termination.
Example:
#include <stdio.h> #include <stdlib.h> int main() { int num; printf("Enter a number: "); scanf("%d", &num); if (num < 0) { printf("Error: Negative number entered. Exiting program...\n"); exit(1); // Exit with non-zero status indicating error } printf("Entered number: %d\n", num); return 0; }
In this example:
- If the user enters a negative number, the program prints an error message and exits with a status of 1 using the
exit(1)
statement. - If the user enters a non-negative number, the program prints the entered number and continues execution normally.
- After calling
exit()
, the program terminates immediately, and any code following theexit()
call is not executed.
Example 1: Program to use the exit() function in the for loop
Let’s create a program to demonstrate the exit (0) function for normal terminating the process in the C programming language.
#include <stdio.h> #include <stdlib.h> int main () { // declaration of the variables int i, num; printf ( " Enter the last number: "); scanf ( " %d", &num); for ( i = 1; i<num; i++) { // use if statement to check the condition if ( i == 6 ) /* use exit () statement with passing 0 argument to show termination of the program without any error message. */ exit(0); else printf (" \n Number is %d", i); } return 0; }
Output
Enter the last number: 10 Number is 1 Number is 2 Number is 3 Number is 4 Number is 5
There are two types of exit status in C
Following are the types of the exit function in the C programming language, as follows:
- EXIT_ SUCCESS
- EXIT_FAILURE
Program to demonstrate the usage of the EXIT_SUCCESS or exit(0) function
Let’s create a simple program to demonstrate the working of the exit(0) function in C programming.
- #include <stdio.h>
- #include <stdlib.>
- int main ()
- {
- printf ( ” Start the execution of the program. \n”);
- printf (” Exit from the program. \n “);
- // use exit (0) function to successfully execute the program
- exit (0);
- printf ( “Terminate the execution of the program.\n “);
- return 0;
- }
Output
Start the execution of the program. Exit from the program.
Example 2: Program to use the EXIT_SUCCESS macro in the exit() function
Let’s create a C program to validate the whether the character is presents or not.
- #include <stdio.h>
- #include <stdlib.h>
- int main ()
- {
- // declaration of the character type variable
- char ch;
- printf(” Enter the character: “);
- scanf (” %c”, &ch);
- // use if statement to check the condition
- if ( ch == ‘Y’)
- {
- printf(” Great, you did it. “);
- exit(EXIT_SUCCESS); // use exit() function to terminate the execution of a program
- }
- else
- {
- printf (” You entered wrong character!! “);
- }
- return 0;
- }
Output
Enter the character: Y
Great, you did it.
Let’s create a program to use the EXIT_FAILURE or exit(1) function
#include <stdio.h> #include <stdlib.h> int main () { int num1, num2; printf (" Enter the num1: "); scanf ("%d", &num1); printf (" \n Enter the num2: "); scanf ("%d", &num2); if (num2 == 0) { printf (" \n Dividend cannot be zero. "); // use EXIT_FAILURE exit(1); } float num3 = (float)num1 / (float)num2; printf (" %d / %d : %f", num1, num2, num3); // use the EXIT_SUCCESS exit(0); }
Output
Enter the num1: 20 Enter the num2: 6 20 / 6 : 3.333333 2nd Run Enter the num1: 20 Enter the num2: 6 Dividend cannot be zero
goto statement
When geto statement is encountered in a program then it transfers the control of the program statements execution unconditionally to the location specified by the goto statement within a current function.
Syntax
goto label;
where the label is an identifier that is used to label the target statement to which the control is transferred. Control may be transferred to anywhere within the current function. The target statement must be labeled, and a colon must follow the label. Thus the target statement will appear as
label: statement;
Each labeled statement within the function must have a unique label, that is, no two statements can have the same label.
//Write a program to display numbers from 1 to 10 using goto statement #include<stdio.h> void main() int i=1; label 1; printf("%d\n"); 1++; if(i<=10) goto label 1; }